Python List Methods and Functions
- Hussam Omari
- 12 فبراير 2023
- 6 دقيقة قراءة
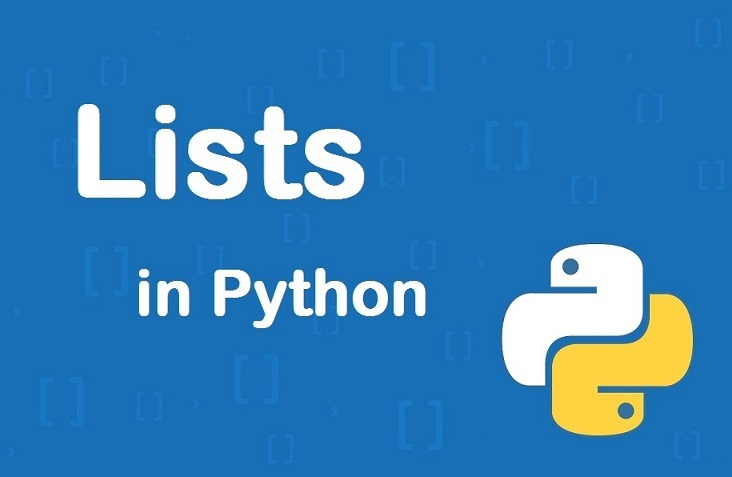
In the previous article, we explain Data Types in Python. Data types are the classification or categorization of data values. It represents the kind of value that tells what operations can be performed on a particular data. In Python, variables can store data of different types including numeric, string, and iterables such as lists, sets, tuples, and dictionaries. The most integral and common iterables in Python are lists which we would explain in this article.
What is a list in Python?
Lists are a containers which can simultaneously store different types of values such as numeric type (e.g. integer and float) and text type (string). Lists in Python can be constructed by placing elements inside square brackets [ ], separated by commas.
Examples:
# empty list:
my_list1 = []
# list of integers:
my_list2 = [9, 18, 30, 15, 18]
# list with mixed data types:
my_list3 = [10, 'j', True, 'Husam']
# nested list:
my_list4 = ['car', [10, 20, 30], ['a']]
It is also possible to use the list() function to create or convert an iterable (a set or tuple) into a list, as shown in the following example:
animals = {"cat", "dog", "parrot"}
# Convert animals set into a list:
newlist = list(animals)
print(newlist)
Output:
['cat', 'dog', 'parrot']
It is worth mentioning that Python does not have built-in support for Arrays, but Python Lists can be used instead. An array is a special variable, which can hold more than one value at a time.
Lists in Python have the following characteristics:
Lists are ordered.
List elements can be accessed by index.
Lists are mutable (changeable / modifiable).
Lists allow duplicate elements.
Accessing elements in Lists
Accessing a Specific Element
List values are indexed and you can access them by referring to the index number. An index number is the number of the position of the value which starts at 0 and must be an integer. For example, a list having 5 elements will have an index from 0 to 4 as illustrated in Figure 1.

Figure 1: List indices
To access a specific value in a list, you need use the variable name of the list followed by the index number of the value placed inside square brackets.
Example:
colors_list = ["red", "blue", "green", "yellow", "pink"]
# accessing the first value:
print(colors_list[0])
# accessing the second value:
print(colors_list[1])
# accessing the last value:
print(colors_list[4])
Outputs:
red
blue
pink
Also, Python allows negative indexing for its sequences. The index of -1 refers to the last item, -2 to the second last item and so on as illustrated in Figure 2.

Figure 2: List negative indexes
Example:
colors_list = ["red", "blue", "green", "yellow", "pink"]
# accessing the first value using negative indexing:
print(colors_list[-5])
# accessing the last value using negative indexing:
print(colors_list[-1])
Outputs:
red
pink
Accessing a Range of Elements
To access a range of values in a list, you need to specify a range of indexes by specifying where to start and where to end the range.
Example:
names = ["Lara", "John", "Sam", "Robert", "Mark"]
# Accessing a range of values:
print(names[0:3])
Outputs:
['Lara', 'John', 'Sam']
Getting Index of an Element
To find the index of an element in a list, we use the index() method. The following example defines a list of cities and uses the index() method to get the index of the element 'London'.
Example:
cities = ['New York', 'Paris', 'London', 'Amman']
result = cities.index('London')
print(result)
Output
2
If you attempt to find an element that does not exist in the list using the index() method, Python will throw an error (ValueError). This means that the item you are searching for does not exist in the list. Such Errors can be handled using try and except statement.
Updating Lists
In Python, lists are mutable. It means we can modify the list’s elements by adding or updating elements from the list. You can use the following methods to modify a list's elements:
Replacing an element with a new value using list indexing
Appending an element to the end of the list using list.append()
Inserting an element before a given index using list.insert()
Extending the list by adding elements of an iterable (list, tuple, string etc.) to the end of the list using list.extend()
List Concatenation using + operator
1. Replacing an Element
You can simply replace an element by using its index number and assign a new value.
Example:
cars = ['Audi', 'Toyota', 'BMW']
# replace first value:
cars[0] = "Mercedes"
print(cars)
Output:
['Mercedes', 'Toyota', 'BMW']
2. append() method
The list.append() method is used to add an element to the end of the list. You can also use this method to add an iterable, such as a list, set, tuple, string and dictionary, into a list.
Example: Add an element (string) to a list
fruits = ['banana', 'apple', 'orange']
# add kiwifruit to the fruits list:
fruits.append("kiwifruit")
print(fruits)
Output:
['banana', 'apple', 'orange', 'kiwifruit']
Example: Add an iterable (list) to another list
list_a = [1, 2, 3]
list_b = [4, 5, 6]
# add list_b to list_a:
list_a.append(list_b)
print(list_a)
Output:
[1, 2, 3, [4, 5, 6]]
3. extend() method
The list.extend() is used to add elements of an iterable, such as a list, set, tuple, string and dictionary, to the end of the list.
Example:
letters_list = ["A", "B", "C"]
numbers_tuple = (1, 2, 3)
# add the tuple to the existing list
letters_list.extend(numbers_tuple)
print(letters_list)
Output:
['A', 'B', 'C', 1, 2, 3]
4. insert() method
The list.insert() is used to add an element at a given index (specified position) of the list. You can also use this method to insert objects (iterables) such as a list, set, tuple, string and dictionary.
Example: Insert an element to the list
laptops = ['Dell', 'Lenovo', 'HP']
# insert 'Sony' at index 2 (3rd position)
laptops.insert(2, 'Sony')
print(laptops)
Output:
['Dell', 'Lenovo', 'Sony', 'HP']
Example: Insert an object to a list
laptops = ['Dell', 'Lenovo', 'HP']
years_set = {2020, 2021, 2022}
# insert years_set at index 3 (4th position)
laptops.insert(3, years_set)
print(laptops)
Output:
['Dell', 'Lenovo', 'HP', {2020, 2021, 2022}]
5. List Concatenation
We can use + operator to concatenate multiple lists and create a new list.
Example:
list_a = [1, 2, 3]
list_b = [4, 5, 6]
# add list_b to list_a:
new_list = list_a + list_b
print(new_list)
Output:
[1, 2, 3, 4, 5, 6]
Deleting List Elements
You can delete a list or remove its elements by using the following methods:
Deleting a list using Python del keyword .
Removing all the elements of a list and make it empty using list.clear().
Removing a specific element using list.remove().
Removes the element at the specified position using list.pop().
1. del keyword
The del keyword in Python can be used to delete objects including variables and iterables (a list, set, tuple, string and dictionary).
Example:
letters = ["A", "B", "C", "D"]
del letters
print(letters)
Output:
NameError: name 'letters' is not defined
Python del keyword can also be used to delete a specific element from the iterable object, or it can also be used to delete a range of elements from the iterables.
Example:
letters_list = ["A", "B", "C", "D", "E", "F", "G"]
# Deleting the first element:
del letters_list[0]
# Deleting a range of elements (E, F, G):
del letters_list[3:6]
print(letters_list)
Output:
['B', 'C', 'D']
2. clear() method
The list.clear() is used to remove all elements of a list and make it empty.
Example:
animals = ["cat", "dog", "parrot"]
#Remove all elements:
animals.clear()
print(animals)
Output:
[]
3. remove() method
The list.remove() is used to remove specific element. If the list contains several similar elements, only the first occurrence of the element is deleted.
Example:
colors = ["Green", "Red", "Red", "Yellow"]
# Remove specific element (Red):
colors.remove("Red")
print(colors)
Output:
['Green', 'Red', 'Yellow']
4. pop() method
The list.pop() method removes and returns the element at a given index (specified position). If no index is specified, Python removes and returns the last item in the list.
Example:
names_list = ["Lara", "John", "Sam", "Robert"]
# Remove and return the second element:
x = names_list.pop(1)
print(x)
Output:
John
Note that using the pop() method on an empty list will raise an error (IndexError).
Modifying the Order of List Elements
In Python, the order of a list elements can be modified using list.sort() and list.reverse() method.
1. sort() method
The list.sort() method is used to sort the list elements. If a list contains numbers, the sort() method sorts the numbers from smallest to largest. If a list contains strings, the sort() method sorts the string elements alphabetically.
Example:
search_engines = ["Google", "Yandex", "Bing", "Ecosia", "OneSearch"]
# List in Ascending Order:
search_engines.sort()
print(search_engines)
Outputs:
['Bing', 'Ecosia', 'Google', 'OneSearch', 'Yandex']
To sort the elements in a list in the reverse alphabetical order, we use reverse=True argument.
# List in Descending Order:
search_engines.sort(reverse=True)
print(search_engines)
Outputs:
['Yandex', 'OneSearch', 'Google', 'Ecosia', 'Bing']
2. reverse() method
The list.reverse() method reverses the positions of the elements in the list. The first element becomes the last element, the second element becomes the second last element, and so on.
Example:
numbers = [2, 4, 3]
# Reverse the positions of the numbers:
numbers.reverse()
print(numbers)
Outputs:
[3, 4, 2]
Other Methods for Lists
1. count() method
The list.count() returns the number of times an element occurs in the list.
Example:
colors = ["red", "red", "blue", "green"]
# Return the number of times "red" occurs in the list
print(colors.count("red"))
Outputs:
2
2. copy() method
The list.copy() returns a copy of the list. The copied list points to a different memory location than the original list, so changing one list does not affect another list.
Example:
names = ["Lara", "John", "Sam", "Robert"]
# create a copy of the names list:
copied_names = names.copy()
print(copied_names)
Outputs:
['Lara', 'John', 'Sam', 'Robert']
3. len() function
You can use the len(list) function to get the length of an iterable. In other words, this function tells us how many elements in a given list, tuple, dictionary, string etc..
Example:
months = ["Jan", "Feb", "March", "April"]
# Return the length of the list:
print(len(months))
Outputs:
4
References
Matthes, E. (2019). Python crash course: A hands-on, project-based introduction to programming: no starch press.
Shovic, J. C., & Simpson, A. (2019). Python All-in-one for Dummies: John Wiley & Sons.
Yates, J. (2016). Python: Practical Python Programming For Beginners and Experts (Beginner Guide): CreateSpace Independent Publishing Platform.
Mueller, J. P. (2014). Beginning programming with python for dummies: John Wiley & Sons.
Comments