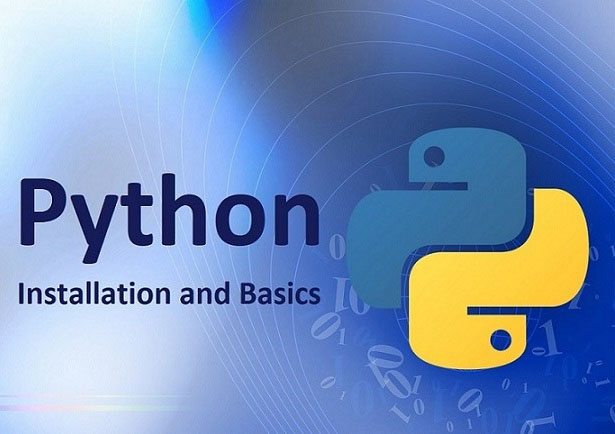
What is Python?
Python is an open-source programming language and one of the most popular and rapidly growing high-level programming languages worldwide. It was created by Guido van Rossum, and released in 1991. It is commonly used for:
Software development
Mathematics and data analysis
Data science and data visualization
Business applications
Machine learning and artificial intelligence
Other uses such as system scripting and web development (server-side)
Python is easy to use and learn and it is a cross-platform programming language, which means it runs on all the major operating systems such as Linux, Windows, Macintosh, and Solaris.
How to Install Python?
Installing Python Directly
Installing python on your operating system (OS) is usually easy. You can download python installer from Python website and then install the file on your OS. The Python download requires about 25 Mb of disk space. When installed, Python requires an additional 90 Mb of disk space. Make sure to mark Add Python 3.10 to PATH otherwise you will have to do it explicitly.
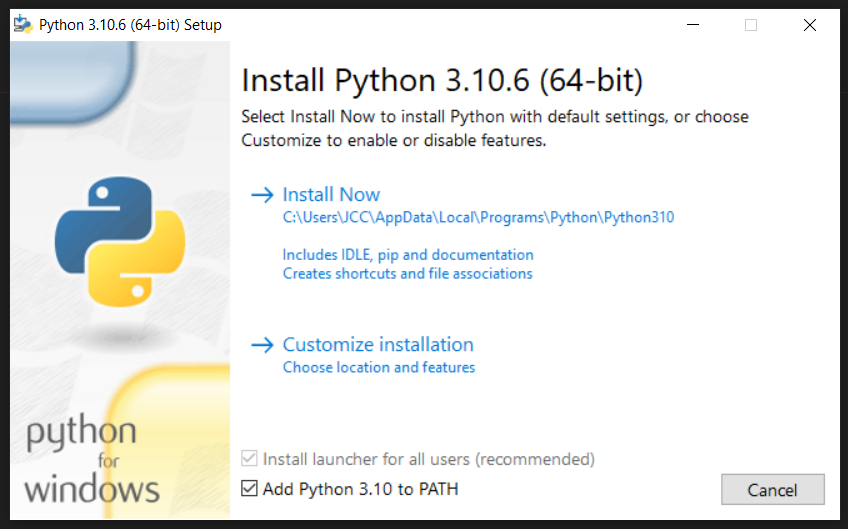
Figure 1: Python installation window
After installation is completed, you can open Python IDLE (Integrated Development and Learning Environment) and start coding. IDLE provides a fully-featured text editor to create Python script that includes features like syntax highlighting, autocompletion, and smart indent. It also has a debugger with stepping and breakpoints features.
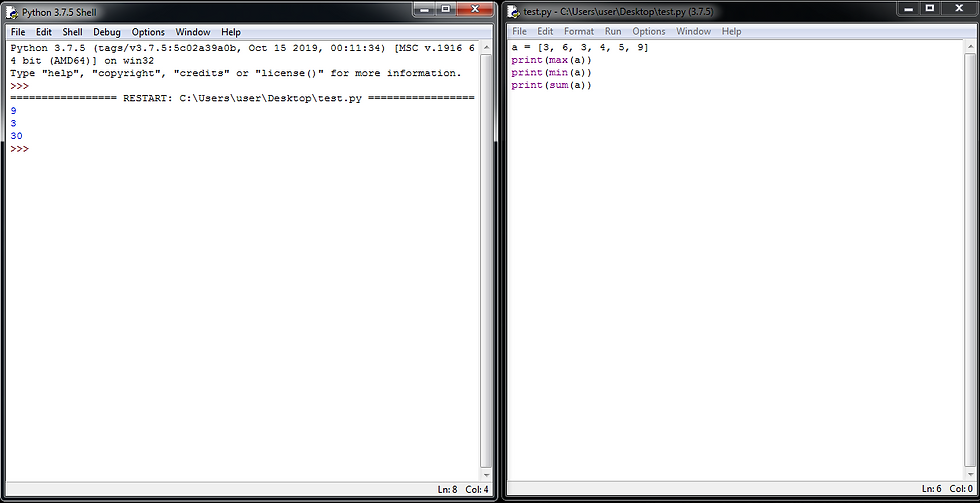
Figure 2: Python IDLE windows
For Linux OS such as Ubuntu, IDLE is not available by default in Python distributions. It needs to be installed using the respective package managers. To install IDLE on Ubuntu, execute the following command:
$ sudo apt-get install idle
You can also install and use other Integrated Development Environments (IDE's) instead of the default Python IDLE such as Visual studio code, Spyder, PyCharm, Sublime, and Atom.
Installing Python With Anaconda
Another more convenient way to install and use Python is through Anaconda which is a data science platform and a complete Python development environment. Anaconda Navigator comes with several IDE's to use such as PyCharm, Spyder and Jupyter. You can easily download and install Anaconda for free from their website.
Note that by installing Anaconda, you do not need to download and install Python directly from Python website as previously mentioned.
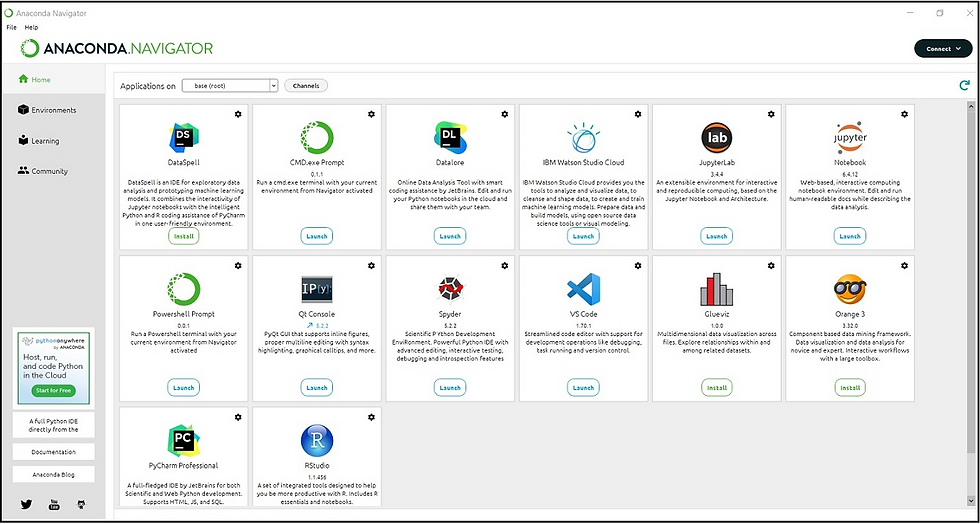
Figure 3. Anaconda platform
For data science and data analysis purposes, it is recommended to use Jupyter Notebook which is included in the Anaconda platform. Jupyter Notebook provides you with an easy-to-use, interactive data science environment. The great feature of Jupyter is that it allows you to segment your code while storing the values of variables from segments you have already run which is also makes it easy to explore and visualize the data.
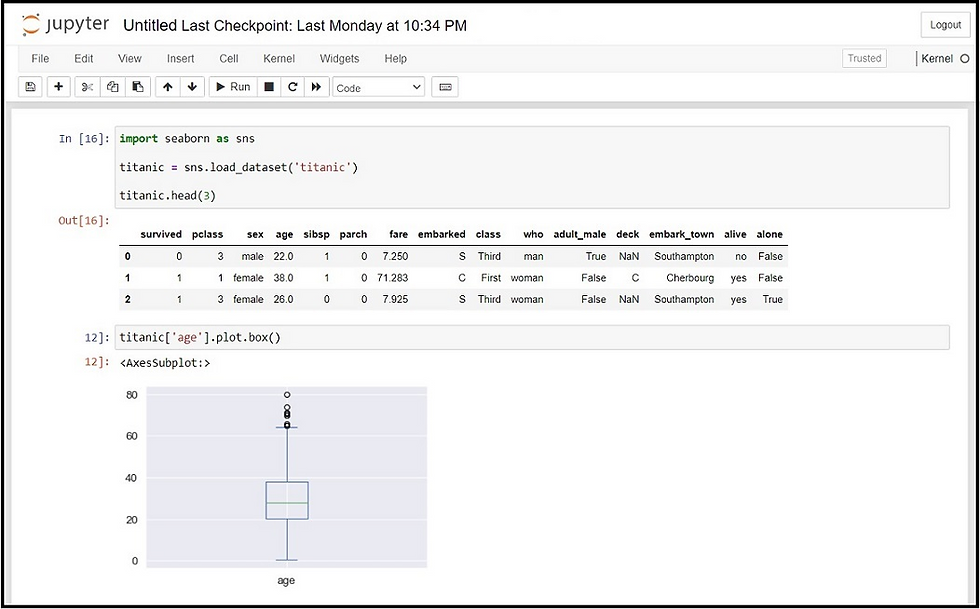
Figure 4: Jupyter Notebook
Python Syntax
The syntax of the Python programming language is the set of rules which defines how a Python program will be written. Python was designed to be a highly readable language; simplified syntax and natural-language flow.
Example:
message = "Hello World"
print(message)
Output:
Hello World
Indentation
The Python language has many similarities to Perl, C, and Java. However, there are some remarkable differences between the languages. The one thing that really makes Python different from other languages is that it uses indentations, rather than parentheses and curly braces, to indicate a chunk or block of code.
Indentation refers to the spaces at the beginning of a code line, as shown in the following Python code:
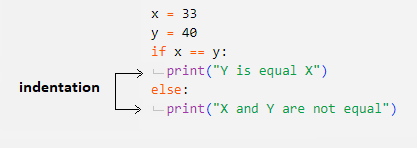
Indentation is a very important concept of Python because without proper indentation, Python does not know which statement to execute next or which statement belongs to which block.
Comments in Python
In computer programming, a programmer comment (usually called a comment for short) is a text note added to source code to provide explanatory information about the function of the code.
Comments are critically important, professionals use comments to explain to team members what their cod is doing. Also, they use comments as notes to themselves to help them review their code in the future.
In python, we write a single line comment by inserting hash character (#) at the beginning of the comment line. Whereas, we write a multi line comments (known as a docstring) by surrounding the comment with triple quotations.
# This is a single line comment
"""
This is a multi line comment known also as a docstring.
it is written in more than just one line using triple
quotation
"""
Python Variables
Variables are containers for storing data values; or a named memory location. The variables python have the following properties:
Do not need to be declared with any particular type.
Must be assigned to a value.
Can change its value.
In python, a variable is created the moment you first assign a value to it. Assignment is done with a single equals sign (=) as illustrated in the following syntax:
variable_name = value
Example:
var1 = 9
var2 = "StatsPlat"
print(var1)
print(var2)
Output:
9
StatsPlat
A variable can have a very short name (such as: x, y, and z), or a more descriptive name (such as: age, myname, total_area). When you write a variable name you need to adhere to the following rules:
A variable name must start with a letter or with underscore character.
A variable name cannot start with a number.
A variable name can only contain alpha-numeric characters (A-Z, 0-9) and underscores.
Variable names are case-sensitive (age, Age and AGE are three different variables).
Keywords are not allowed in Python, such as: if, for, while, def, except, class, etc.
Python Operators
Operators are standard symbols used to perform logical and arithmetic operations on values and variables. Python divides the operators in the following groups:
Arithmetic operators
Comparison operators
Assignment operators
Logical operators
Identity operators
Membership operators
Bitwise operators
Arithmetic operators
Arithmetic operators are used to performing mathematical operations like addition, subtraction, multiplication, and division.
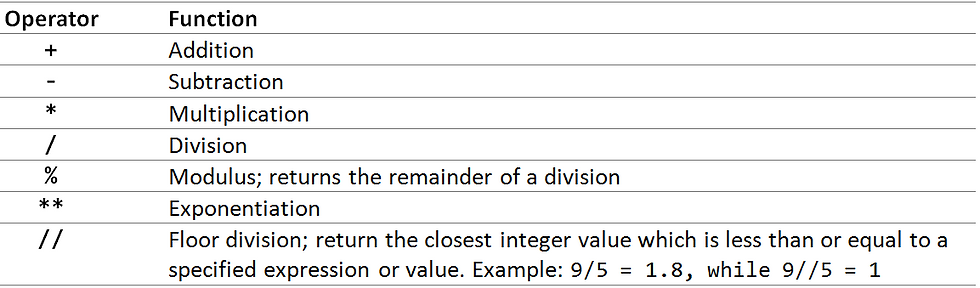
Comparison Operators
Comparison operators compares the values. It either returns True or False according to the condition.
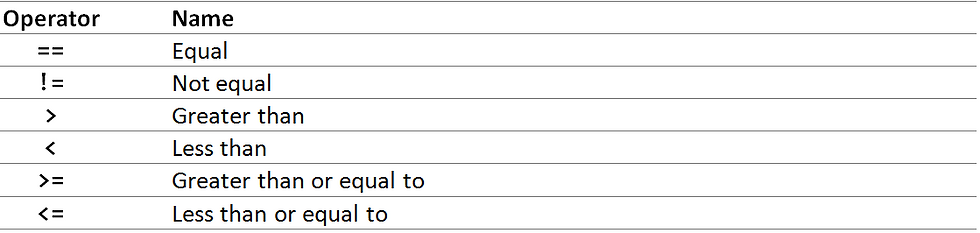
Logical Operators
Logical operators are used to combine conditional statements:

Identity Operators
Identity operators are used to check if two values are the same object and located on the same memory location. Two variables that are equal do not imply that they are identical.

Membership Operators

Bitwise Operators
In Python, bitwise operators are used to perform bitwise calculations on integers. The integers are first converted into binary and then operations are performed on bit by bit, hence the name bitwise operators. Then the result is returned in decimal format.
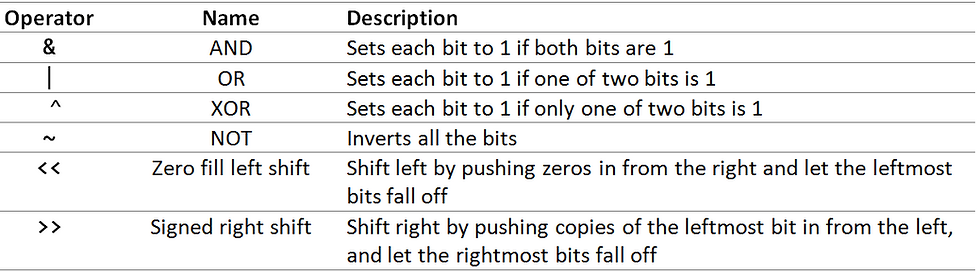
References
Matthes, E. (2019). Python crash course: A hands-on, project-based introduction to programming: no starch press.
Mueller, J. P. (2014). Beginning programming with python for dummies: John Wiley & Sons.
Shovic, J. C., & Simpson, A. (2019). Python All-in-one for Dummies: John Wiley & Sons.
Yates, J. (2016). Python: Practical Python Programming For Beginners and Experts (Beginner Guide): CreateSpace Independent Publishing Platform.
Comments