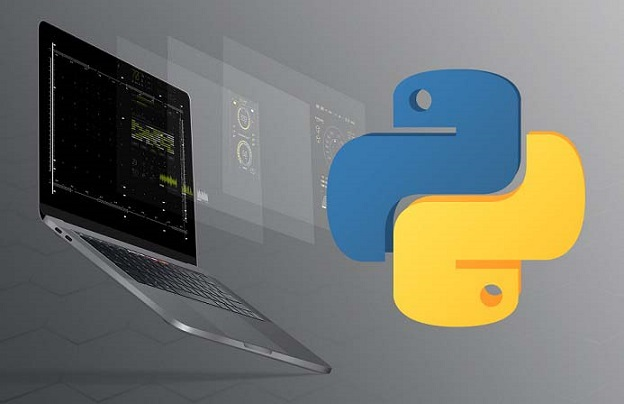
Data Types in Python
Every value in Python has a data type. Data types are the classification or categorization of data values. It represents the kind of value that tells what operations can be performed on a particular data. In Python, variables can store data of different types, as shown in the following table:
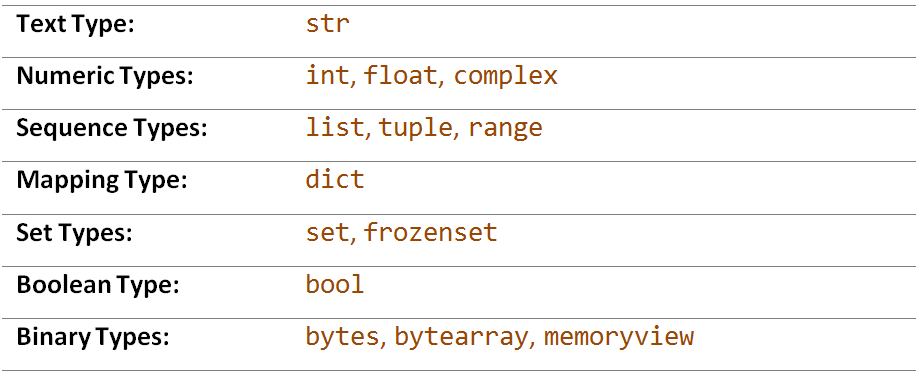
1. Text Type (String)
In Python, strings are sequences of Unicode characters surrounded by a single quote, double-quote or triple quote.
Example:
string1 = "This is a single line string."
string2 = """This is a multi line string surrounded
by triple quotations."""
print(string1)
print(string2)
Output:
This is a single line string.
This is a multi line string surrounded
by triple quotations.
2. Numeric Types
In Python, numeric data type represents the data that has numeric value. A numeric value can be an integer, floating or complex number.
Integers include positive or negative whole numbers (without fraction or decimal).
Float is a real number with floating point representation. It is specified by a decimal point. Optionally, the character e or E followed by a positive or negative integer may be appended to specify scientific notation.
Complex number is represented by complex class. It is specified as (real part) + (imaginary part).
Example:
a = 5 # 5 is an integer
b = 5.2 # 5.2 is a float number
c = 2+3j # 2+3j is a complex number
print(type(a))
print(type(b))
print(type(c))
Output:
<class 'int'>
<class 'float'>
<class 'complex'>
3. Sequence Types
In Python, a sequence is the collection of similar or different data types. Sequences allows us to store multiple values in an organized and efficient fashion such as Lists and Tuples.
Lists in Python
Lists are a containers which can simultaneously store different types of values such as numeric type and text type (string). A list in python is ordered, changeable and indexed (can be accessed by using the method of Indexing); each value in a list has an index number, which tells us the position of the element in that list. Lists also allow duplicate elements.
Lists can be constructed by placing elements inside square brackets [ ], separated by commas.
Example:
# empty list:
my_list1 = []
# list of integers:
my_list2 = [10, 20, 30]
# list with mixed data types:
my_list3 = [10, 'j', True, 'Husam']
# nested list:
my_list4 = ['car', [10, 20, 30], ['a']]
Note that Python does not have built-in support for Arrays, but Python Lists can be used instead. An array is a special variable, which can hold more than one value at a time.
Tuples in Python
Similar to lists, tuples are used to store multiple elements in a single variable. Tuple elements are also ordered, indexed and allow duplicate values. But Tuples are unchangeable, meaning that we cannot change, add or remove elements after the tuple has been created. A tuple can be created by placing all elements inside parentheses ( ), separated by commas.
Examples:
# Tuple having integers:
my_tuple1 = (1, 2, 3)
# tuple with mixed datatypes:
my_tuple2 = (1, "hello", 4.6)
# nested tuple:
my_tuple3 = (1, "hello", [8, 4, 6], (1, 2, 3))
4. Mapping Type (Dictionary)
Dictionaries are used to store data values in key:value pairs. A dictionary elements are ordered, indexed (can be accessed using keys), changeable and does not allow duplicates for keys. A dictionary can be created by placing all elements inside curly brackets { }, separated by commas.
Examples:
# empty dictionary
my_dict1 = {}
# dictionary with integer keys
my_dict2 = {1: 'apple', 2: 'banana'}
# dictionary with mixed keys
my_dict3 = {'name': 'Husam', 'age': 35}
5. Set Types
A set is a collection of unique elements. The set’s elements are unordered, unchangeable, and unindexed. Also, duplicate are not allowed, but we can add new elements. We use the curl brackets { } to construct sets.
Examples:
my_set1 = {1, 2, 3}
my_set2 = {1.0, "Hello", (1, 2, 3)}
my_set3 = {1, 2, 3, 4, 4} # one of the duplicate (4) will be ignored
print(my_set1)
print(my_set2)
print(my_set3)
Outputs:
{1, 2, 3}
{1.0, (1, 2, 3), 'Hello'}
{1, 2, 3, 4}
6. Boolean Type
Boolean type represents one of the two values (True / False) which is used to evaluate values and variables. For instance, 1==1 is True whereas 2<1 is False.
Example:
print(10 > 9)
print(10 == 9)
print(10 < 9)
Output:
True
False
False
7. Binary Types
Bytes and bytearray are used for manipulating binary data. Binary data is a type of data that is represented or displayed in the binary numeral system. Binary data is the only type of data that can be directly understood and executed by a computer. It is numerically represented by a combination of zeros and ones.
The memoryview uses the buffer protocol to access the memory of other binary objects without needing to make a copy.
Example:
x = b"StatsPlat"
y = bytearray(6)
z = memoryview(bytes(6))
print(x)
print(y)
print(z)
Output:
b'StatsPlat'
bytearray(b'\x00\x00\x00\x00\x00\x00')
<memory at 0x00000234960DD580>
Python type() Function
We can use the type() function to know which class a variable or a value belongs to. Similarly, the isinstance() function is used to check if an object belongs to a particular class.
Example:
my_number = 15
my_text = "StatsPlat"
print(type(my_number))
print(type(my_text))
Output:
<class 'str'>
Python Type Casting
Casting in Python is simply a method to convert the variable data type into a certain data type in order to the operation required to be performed by users. Mainly, casting can be done with the following data type function:
Int() : This function take float or string as an argument and return integer (int) type object.
float() : This function take int or string as an argument and return float type object.
str() : This function take float or int as an argument and return string type object.
Example:
x1 = 5.2 # 5.2 is a float type
x2 = int(x1) # convert 5.2 into integer type
x3 = str(x1) # convert 5.2 into string type
print(x1,type(x1))
print(x2,type(x2))
print(x3,type(x3))
Output:
5.2 <class 'float'>
5 <class 'int'>
5.2 <class 'str'>
References
Matthes, E. (2019). Python crash course: A hands-on, project-based introduction to programming: no starch press.
Shovic, J. C., & Simpson, A. (2019). Python All-in-one for Dummies: John Wiley & Sons.
Yates, J. (2016). Python: Practical Python Programming For Beginners and Experts (Beginner Guide): CreateSpace Independent Publishing Platform.
Mueller, J. P. (2014). Beginning programming with python for dummies: John Wiley & Sons.
Comments